Tech Blog
Protected: Vue.js Notebook
Fix PostGreSQL Database Hung During Drop or Alter Tables
You may find yourself in a situation where Postgres decides to stop responding after an Alter or Drop command was given in PGAdmin. This can be corrected by using the following commands below.
Shell into Postgres:
psql
Select data from the pg_stat_activity table.
SELECT pid, now() - pg_stat_activity.query_start AS duration, query, state FROM pg_stat_activity WHERE (now() - pg_stat_activity.query_start) > interval '5 minutes';
Kill the zombie process after getting the PID exit Postgres and run:
sudo kill -9 <ProcessID>
Adding Buefy Autocomplete Quick Start
Input box in <template>:
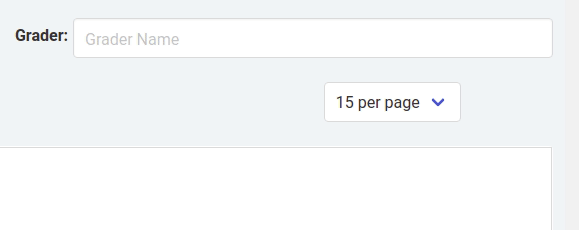
Input box in <template>:
<b-autocomplete v-model="testGrader" placeholder="Grader Name" :data="filteredDataArray" > </b-autocomplete>
Created:
created() { this.getAllGraders(); this.filteredGraders(); },
Computed:
filteredDataArray() { return this.gradersArray.filter(option => option .toString() .toLowerCase() .indexOf(this.testGrader.toLowerCase()) >= 0); },
Data:
data() { return { graderList: [], gradersArray: [], }; }
Method (Get Data From Store):
methods: { getAllGraders() { this.graderList = this.$store.state.graders; }, filteredGraders() { this.graderList.forEach((data) => { if (data !== null) { this.gradersArray.push(data); } }); }, }
Sample data from $store:
[null,"Madge Calbreath","Jacquenette Romera","Frazier Harvison","Kennith Perford","Flory Castiglione","Kerri Fedoronko","Beauregard Northgraves","Terrel Giraths","Kenton Lickorish","Egbert Mallya","Axel Aldwich","Bria Rames","joe","Rhoda Lordon","Durant Shalliker","Christophorus Woodhouse","Creight Lennox","Gardiner Lauret","Rasia MacConneely","Shelby Antoniewski"]
OpenShift Commonly Used Commands
Login:
oc login <urlWithToken>
Select project/namespace:
oc project <projectname>
Get all pods in a project
oc get pods
Port Forward a pod to localhost
oc port-forward <pod>
View logs of a pod
oc logs <pod>
Show status of a current project
oc status
Add users to namespace/project
oc adm policy add-role-to-group edit ocp_devs -n <project-namespace>
Copy File To Pod/Container
oc rsync <source> <destination>
Import Images to OpenShift
oc import-image <image> --from=<docker.example.com/org/image> --confirm
Image Stream Triggers (Mitch Murphey)
oc import-image <org>/<image>:<tag> --from=<docker.example.com/org/image> --confirm oc new-app --docker-image=<org>/<image>:<tag> oc set triggers dc/<image> --from-image=<org>/<image>:<tag> --auto=True -c=<image>
Link builder secrets (env)
oc secrets link builder openshift-private-ssh
oc new-app nodejs~git@gitlab.agilesof.com:esof/esof-fe-clientside.git --source-secret openshift-private-ssh --name fe-clientside
Quick Guide – Setting Up Git For GitLab
Create a SSH Key:
$ ssh-keygen -o -t rsa -b 4096 -C "email@example.com"
Navigate to ssh directory.
$ cd .ssh/
Concatenate your public key for GitLab
$ cat id_rsa.pub
Navigate to ssh directory.
Copy all the output [DO NOT CLOSE THIS WINDOW]:
For example (Don’t copy this code): ssh-rsa AAAAB3NzaC1yc2EAAAADAQABAAACAQCoXdbhi4Lic250qAnuFYx2p5u7pxdGV/0yOxBan2OMlGVkIQWG6WwgZ9awe4wLa7k8DhHT87AeguogKlcdw3WBJMXSVH6OebIkk0LtsewM2ovRCvwg6rEp6xuOOlmpC2RGehrnKYIet/LQXrhAEVZAo5mJo9s+6wUdd5belNuL2ygiF2SQOSTepGuuVkvLmiBihPatld/M89W8fA866doBVyxk0nJzhlsdefYQIt8Ifqd622FbY6G11IQsDyG/lQj0zat3abgEv/CExW4e/+f1XycTCR/lFDLVWviur/CtcpchMWti8jDK2Tb/v5VK0LLkUNLyHcyOvc4zaA6JSz18quYGpcoDM/qvCgZ5jyPoW1GOugKdg9SaqstdJpo6L0adCURi/BVp7cjW3YSTob3ogKspgC7IKWds2DaceeCEg+LYhn9wVapTJCu4bT/r0v9lM7FjPde58QXGqJBvu76AdQs4zXges8+tSkafYfeis3WtFLsLcT1UhlbivC4x9S1Iy9Xp0Lesw2YWSkPTSXTsdziE6WjI7dSJ5DEMWV8E8un2FmsLcozf5ewy9sXls1IxfgJSngAKqOsWdCiGpEv5RFPKbjAcABWqYZ6As0b0R8JxF1hjgihLa1DTiAX2wnxaCgFRO62UJm4m3JmG/JCdMS2lNoLJs1CHvrkOZB72Q== Your.Email@Here.com
Upload your key to Gitlab by logging into your account and clicking your profile icon in the top the right corner, and click Settings then SSH Keys.
Next, you need to tell your local git that you have an active ssh directory, so while you are still in the .ssh directory type in:
$ pwd
Copy the value that is output by that command.
$ git config --system http.SSLCAPath <yourSSHDirectory>
Also if you are working in Windows Powershell Environment you may need to run the following command for Git to use SChannel, the built-in Windows networking layer.
$ git config --global http.sslBackend schannel